What is Redux? Why Use Redux With React for Front-end Web Development?
For beginners, Redux might seem like anything from a library to a state management tool. To simplify matters, Redux is a JavaScript framework for state management. So, what is state management, and what does it mean when one says React Redux together? Let’s start from scratch.
A state is an object containing the original data along with its modifications. Managing the state, or state management, involves keeping track of changes in data as it is shared across different components of the application. This process tracks the data flow until its latest state.
Now that we understand that Redux can maintain a complete data-flow architecture for apps, it’s fair to say that Redux can be used with any framework designed for front-end web application development, such as React JS, Angular JS, Angular, Ember, or Vue.
What is Redux in the React context?
React JS is essentially a front-end web development toolkit that has built-in functions for components to manage their state. This feature dismisses the need to integrate with any external state management tool. However, React JS has a straightforward data-flow architecture that limits the flow of data among different components to a single direction. The more components there are, the more complicated the data-sharing and retrieval process becomes. Why is that?
Ideally, when data needs to be shared in React JS, a method is passed as props by the parent component to the sub-components to update the state information. The state then lives within that sub-component. However, sharing the state among sibling components (sidewise) is not possible in React.
Therefore, the state must live at the app level to enable the downward flow of data.
But here’s the catch:
When the state travels downwards to the components that need it, it passes through all components that do not need that data. This process makes the data flow less predictable due to potential inaccuracies in reasoning. Hence, managing the state within React can become increasingly complex.
This is Where Redux Steps In
Redux is a predictable state management library that centralizes the state of your application into a single source of truth, known as the store. By doing so, Redux allows you to manage the state across your entire application in a way that is consistent, predictable, and easy to debug. Instead of passing the state down through multiple components, Redux enables direct access to the state wherever it’s needed, thus simplifying data management and improving the scalability of your React application.
How does Redux work?
By incorporating Redux, the issue of placing the data at the top component is resolved. The data can exist as a separate entity altogether with Redux, and the required data can be pulled out with a specific code snippet for that component.
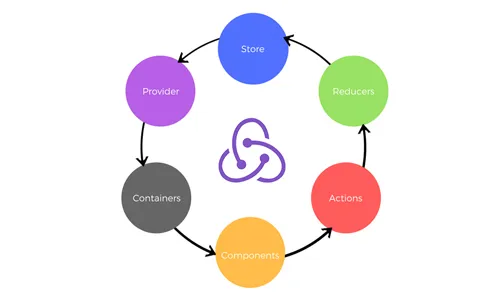
But that’s not all. In addition to keeping the app’s state (changes in data) in one place, Redux also enables tracking the changes with proper reasoning, making it predictable. This predictability is why Redux is referred to as a “predictable state container.”
The Three Core Concepts of Redux
Redux handles state management in apps through three key concepts: Actions, Reducers, and Store.
Actions
Actions are methods in Redux that deposit data collected from user interactions, form submissions, or API interfaces into the Redux store.
The store.dispatch()
function is a JavaScript object associated with a type
property. The action type could be anything, such as login or search, depending on the component for which the data needs to be stored in the container. Action creators are functions that encapsulate the action object creation process and return action objects.
const setLoginStatus = (username, password) => {
return {
type: "LOGIN",
payload: {
username: username,
password: password
}
};
};
Reducers
Reducers are intermediary methods that consider the current state of the application, perform an action on it, and then create a new state. The job of reducers is carried out via the reducer()
function in JavaScript, which returns objects based on each action performed on the store.
const LoginComp = (state = initState, action) => {
switch (action.type) {
// This reducer handles all actions with type “LOGIN”
case "LOGIN":
return state.map(user => {
if (user.username !== action.username) {
return user;
}
if (user.password === action.password) {
return {
...user,
login_status: "LOGGED IN SUCCESSFULLY"
};
}
return user;
});
default:
return state;
}
};
Store
The store methods like createStore()
essentially store the state of the application. There is a single store for a Redux application, accessible by all the components of the app. For every action triggered in Redux, a new state is created. This state is then carried forward to store, update, and delete data through helper methods at various stages. This streamlined data flow in Redux makes the state more traceable and predictable.
// Redux Store Creation
const store = createStore(LoginComp);
// React Component
class MyApp extends React.Component {
render() {
return (
<div>
<Status user={this.props.user.name} />
<Login login={this.props.setLoginStatus} />
</div>
);
}
}
Advantages of using React Redux
When building complex front-end applications with React, managing the state across numerous components can become challenging. This is where Redux comes into play, offering several advantages that make state management more efficient and predictable. Let’s explore the key benefits of using React Redux in your web development projects.
1. Centralized State Management
One of the primary advantages of Redux is its centralized state management. In a React application, the state is typically managed at the component level. However, as the application grows, this approach can lead to scattered and hard-to-track states. Redux resolves this by storing the entire application state in a single, centralized store. This ensures that every component has access to the state it needs without the need for prop drilling or cumbersome state lifting.
Benefits:
- Ease of Access: Any component in the app can access the state it needs directly from the store.
- Simplified Debugging: With the entire state in one place, tracking changes and identifying issues becomes easier.
2. Predictable State Changes
Redux enforces strict rules on how and where the state can be changed. This predictability is achieved through the use of pure functions known as reducers, which are responsible for handling state changes based on actions. Since reducers always return a new state rather than mutating the existing one, it becomes straightforward to predict the outcome of a particular action.
Benefits:
- Consistency: State changes follow a clear and consistent pattern, reducing the likelihood of unexpected bugs.
- Time Travel Debugging: Redux’s predictable state management enables advanced debugging techniques like time travel, where you can step back and forth through state changes to identify the source of an issue.
3. Enhanced Debugging and Development Tools
Redux offers a suite of powerful development tools, such as the Redux DevTools Extension, which integrates seamlessly with your browser. These tools provide a real-time view of the state, actions, and state changes, making it easier to debug and optimize your application.
Benefits:
- Real-Time Insights: Monitor state changes, actions, and dispatch events as they happen in real time.
- State Snapshots: Capture and review state snapshots to understand the application’s behavior at any given point.
4. Simplified Data Flow
In a React application, data flow can become complex, especially when components need to share state. React follows a unidirectional data flow, meaning data is passed down from parent to child components via props. Redux simplifies this by allowing any component to access the global state directly from the store, eliminating the need for complex data flows and reducing the risk of errors.
Benefits:
- Reduced Prop Drilling: No need to pass props through multiple layers of components just to share data.
- Direct Access: Components can pull only the data they need from the store, leading to cleaner and more maintainable code.
5. Improved Code Maintainability
With Redux, the state management logic is separated from the UI logic, leading to a more modular codebase. Actions, reducers, and store configurations are kept distinct, making it easier to maintain, scale, and test the application as it grows.
Benefits:
- Modularity: Separate concerns by keeping state management logic independent of UI components.
- Scalability: Easily scale the application by adding new features without entangling state management with the component logic.
6. Better Collaboration and Team Workflow
In larger teams, Redux’s clear structure and centralized state management can improve collaboration. Developers can work on different parts of the application without stepping on each other’s toes, as the state logic is centralized and well-documented.
Benefits:
- Clear Responsibilities: Teams can work independently on actions, reducers, and components, improving productivity.
- Consistent Standards: Redux enforces consistent patterns for state management, making it easier for team members to understand and contribute to the codebase.
7. Compatibility with React Ecosystem
Redux is highly compatible with the React ecosystem and integrates well with other popular libraries and tools. Whether you’re using React Router, middleware like Redux Thunk, or handling side effects with Redux Saga, Redux offers a flexible and robust foundation for building complex applications.
Benefits:
- Seamless Integration: Easily integrate Redux with other React libraries and tools.
- Extensibility: Add middleware and plugins to extend Redux’s capabilities as your application grows.
Conclusion
React Redux offers a powerful and efficient solution for managing the state in complex front-end applications. By centralizing the state, enforcing predictable state changes, and providing robust development tools, Redux simplifies the process of building and maintaining scalable React applications. As you continue to develop your projects, leveraging the advantages of Redux can lead to cleaner code, better performance, and a more enjoyable development experience.
FAQs on Redux in React: Functions and Advantages
1. What is Redux and why use it with React?
Redux is a JavaScript library for state management, crucial for managing complex states in React applications. It centralizes the application state, making data flow more predictable and manageable across components.
2. How does Redux work with React?
Redux works by maintaining a single source of truth (store) for the entire application state. Actions trigger state changes through reducers, which update the store. Components subscribe to the store to access and update state data efficiently.
3. What are the benefits of using Redux in React?
Redux simplifies state management by eliminating the need for prop drilling and lifting state. It enhances predictability with a unidirectional data flow, improves debugging with a centralized store, and supports time-travel debugging and middleware for asynchronous operations.
4. What are Redux actions, reducers, and stores in React?
Actions are payloads of information that send data from your application to the Redux store. Reducers specify how the application’s state changes in response to actions. The store holds the state of your application.
5. What are the drawbacks of using Redux in React applications?
While Redux offers significant benefits, it can introduce complexity to smaller applications. Setting up Redux requires boilerplate code, and overusing Redux for simpler state management can lead to unnecessary complexity in codebase maintenance.